Saving the resized images
Last article concluded with us creating a web role that will retrieve an image from blob storage, resize it, raise an event, and stream the result back.
This article is about the worker role to handle those raised events.
Simply enough, all we’ll be doing is creating a worker role, hooking into the same azure service bus queue, picking up each message, pulling out the relevant data within, and uploading that to blob storage.
Overall Process
A reminder of the overall process:
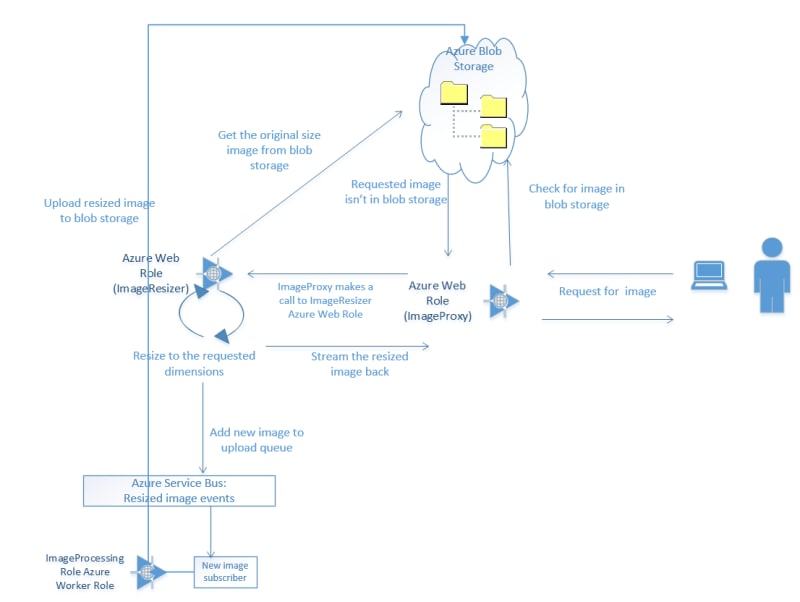
The Worker Role
The section of that which the worker role is responsible for is as below:
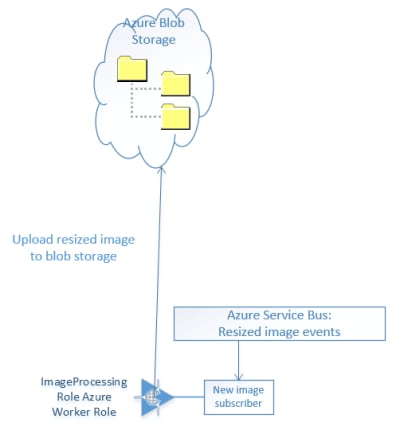
Add a new worker role to the Cloud project within the solution from last time (or a new one if you like). This one consists of four little methods; Run, OnStart, and OnEnd, where Run will call an UploadBlob method.
Run
This method will pick up any messages appearing on the queue, deserialize the contents of the message to a known structure, and pass them to an uploading method.
Kick off by pasting over the Run method with this one, including the definitions at the top – set the QueueName to the same queue you configured for the resize notification from the last post:
[csharp] const string QueueName = "azureimages";
QueueClient _client;
readonly ManualResetEvent _completedEvent = new ManualResetEvent(false);
public override void Run()
{
_client.OnMessage(receivedMessage =>
{
try
{
// Process the message
var receivedImage = receivedMessage.GetBody<ImageData>();
UploadBlob("resized", receivedImage);
}
catch (Exception e)
{
Trace.WriteLine("Exception:" + e.Message);
}
}, new OnMessageOptions
{
AutoComplete = true,
MaxConcurrentCalls = 1
});
_completedEvent.WaitOne();
}
[/csharp]
Yes, I’m not doing anything with exceptions; that’s an exercise for the reader.. ahem… (Me? Lazy? Never..happypathhappypathhappypath)
Naturally you’ll get a few squiggles and highlights to fix; Install-Package Microsoft.ServiceBus.NamespaceManager will help with some, as will creating the stub UploadBlob.
Now, to tidy up the reference to ImageData you could do a few things:
- Copy the ImageData.cs over from the previous project into this one
- Create a reference to the previous project and add in a using to this file
-
Extract ImageData from the previous project into a common referenced project for them both to share.
I can live with my own conscience, so am just whacking in a reference to the previous project. Don’t hate me.
OnStart and OnStop
[csharp] public override bool OnStart()
{
// Set the maximum number of concurrent connections
ServicePointManager.DefaultConnectionLimit = 2;
// Create the queue if it does not exist already
var connectionString = CloudConfigurationManager.GetSetting("Microsoft.ServiceBus.ConnectionString");
var namespaceManager = NamespaceManager.CreateFromConnectionString(connectionString);
if (!namespaceManager.QueueExists(QueueName))
{
namespaceManager.CreateQueue(QueueName);
}
// Initialize the connection to Service Bus Queue
_client = QueueClient.CreateFromConnectionString(connectionString, QueueName);
return base.OnStart();
}
public override void OnStop()
{
// Close the connection to Service Bus Queue
_client.Close();
_completedEvent.Set();
base.OnStop();
}
[/csharp]
OnStart gets a connection to the service bus, creates the named queue if necessary, and creates a queue client referencing that queue within that service bus.
OnStop kills everything off.
So, off you pop and add the requisite service connection string details; right click the role within the cloud project, properties:
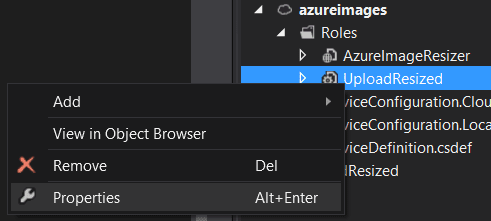
Click settings, add setting “Microsoft.ServiceBus.ConnectionString” with the value you used previously.
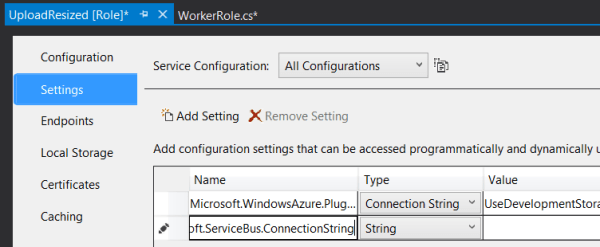
Lastly:
UploadBlob
[csharp] public void UploadBlob(string path, ImageData image)
{
var connectionString = CloudConfigurationManager.GetSetting("Microsoft.Storage.ConnectionString");
var account = CloudStorageAccount.Parse(connectionString);
var cloudBlobClient = account.CreateCloudBlobClient();
var cloudBlobContainer = cloudBlobClient.GetContainerReference(path);
cloudBlobContainer.CreateIfNotExists();
var blockref = image.FormattedName ?? Guid.NewGuid().ToString();
var blob = cloudBlobContainer.GetBlockBlobReference(blockref);
if (!blob.Exists())
blob.UploadFromStream(new MemoryStream(image.Data));
}
[/csharp]
Pretty self explanatory, isn’t it? Get a reference to an area of blob storage within a container associated with an account, and stream some data to it if it doesn’t already exist (you might actually want to overwrite it so could remove that check). Bosch. Done. Handsome.
Notice we’re using the FormattedName property on ImageData to get a blob name which includes the requested dimensions; this will be used in the next article where we create the image proxy.
This means that for a request like:
[csharp]http://127.0.0.1/api/Image/Resize?height=600&width=400&source=image1.jpg
[/csharp]
The formatted name will be set to:
[csharp]600_400-image1.jpg
[/csharp]
You shouldn’t get any compile errors here but you’ll need to add in the setting for your storage account (“Microsoft.Storage.ConnectionString”).
Kick it off
To run that you’ll need VS to be running as admin (right click VS, run as admin):
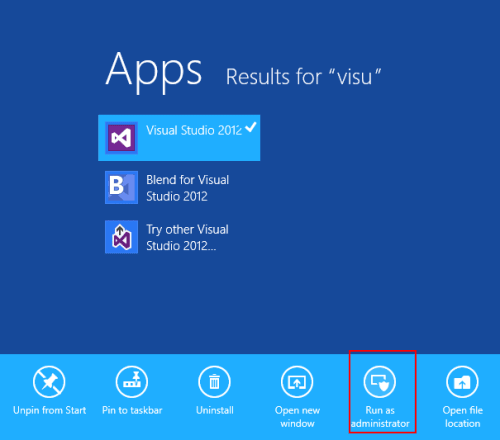
After you’ve got it running, fire off a request within the resizing web api (if it’s not the same solution/cloud service) for something like:
[csharp]http://127.0.0.1/api/Image/Resize?height=600&width=400&source=image1.jpg
[/csharp]
Resulting in:
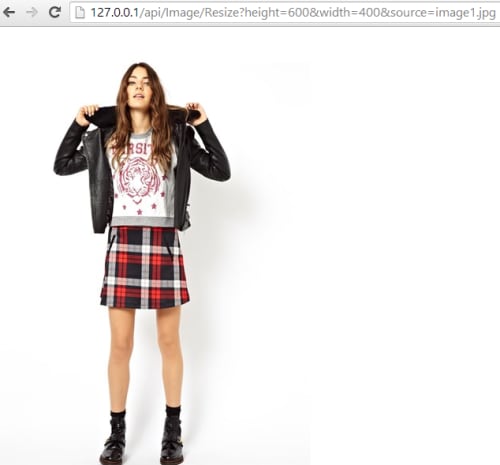
Then open up your Azure storage explorer to see something similar to the below within the “resized” blob container:
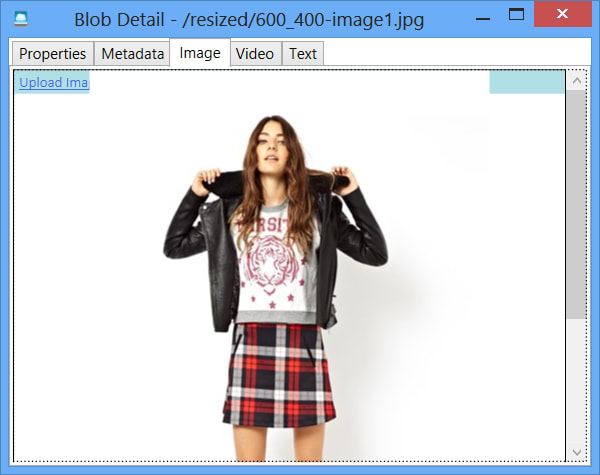
What happened?
- The ImageController on your Resizer Web API web role did the hard work and popped a message on an Azure Service Bus queue containing the image data
- The new Uploader worker role is subscribed to the same Azure Service Bus queue
- it picks up the message
- pulls out the image data
- generates an image name based on the image dimensions and origin
- streams the image data into a blob block with the generated name
Cool, huh?
The code for this series is up on GitHub
Next up
One more web role to act as a proxy for checking blob storage first before firing off the resize request. Another easy one. Azure is easy. Everyone should be doing this. You should wait and see what else I’ll write about Azure.. it’s awesome.. and easy..!